Google ADs
ANSWERS @https://ipwithease.com/shop/
- Difference between Array and Array List.
- What is the result of the following class?
1: public class _C {
2: private static int $;
3: public static void main(String[] main) {
4: String a_b;
5: System.out.print($);
6: System.out.print(a_b);
7: } } - What is the result of the following code?
String s1 = “Java”;
String s2 = “Java”;
StringBuilder sb1 = new StringBuilder();
append(“Ja”).append(“va”);
System.out.println(s1 == s2);
System.out.println(s1.equals(s2));
System.out.println(sb1.toString() == s1);
System.out.println(sb1.toString().equals(s1)); - Differences between HashMap and HashTable in Java.
- What is meant by Collections in Java?
- What is the output of the following code?
1: interface HasTail { int getTailLength(); }
2: abstract class Puma implements HasTail {
3: protected int getTailLength() {return 4;}
4: }
5: public class Cougar extends Puma {
6: public static void main(String[] args) {
7: Puma puma = new Puma();
8: System.out.println(puma.getTailLength());
9: }
10:
11: public int getTailLength(int length) {return 2;}
12: } - What is the output of the following program?
1: public class FeedingSchedule {
2: public static void main(String[] args) {
3: boolean keepGoing = true;
4: int count = 0;
5: int x = 3;
6: while(count++ < 3) {
7: int y = (1 + 2 * count) % 3;
8: switch(y) {
9: default:
10: case 0: x -= 1; break;
11: case 1: x += 5;
12: }
13: }
14: System.out.println(x);
15: } } - What is the output of the following code snippet?
1: System.out.print(“a”);
2: try {
3: System.out.print(“b”);
4: throw new IllegalArgumentException();
5: } catch (RuntimeException e) {
6: System.out.print(“c”);
7: } finally {
8: System.out.print(“d”);
9: }
10: System.out.print(“e”); - What is the result of the following program?
1: public class MathFunctions {
2: public static void addToInt(int x, int amountToAdd) {
3: x = x + amountToAdd;
4: }
5: public static void main(String[] args) {
6: int a = 15;
7: int b = 10;
8: MathFunctions.addToInt(a, b);
9: System.out.println(a); } } - What is the result of the following code?
1: int[] array = {6,9,8};
2: List<Integer> list = new ArrayList<>();
3: list.add(array[0]);
4: list.add(array[2]);
5: list.set(1, array[1]);
6: list.remove(0);
7: System.out.println(list); - What is the output of the following code?
1: public class Deer {
2: public Deer() { System.out.print(“Deer”); }
3: public Deer(int age) { System.out.print(“DeerAge”); }
4: private boolean hasHorns() { return false; }
5: public static void main(String[] args) {
6: Deer deer = new Reindeer(5);
7: System.out.println(“,”+deer.hasHorns());
8: }
9: }
10: class Reindeer extends Deer {
11: public Reindeer(int age) { System.out.print(“Reindeer”); }
12: public boolean hasHorns() { return true; }
13: } - Which exceptions should be caught?
- Which objects are ready for garbage in the following code?
1: import java.util.*;
2: public class Grasshopper {
3: public Grasshopper(String n) {
4: name = n;
5: }
6: public static void main(String[] args) {
7: Grasshopper one = new Grasshopper(“g1”);
8: Grasshopper two = new Grasshopper(“g2”);
9: one = two;
10: two = null;
11: one = null;
12: }
13: private String name; } - What is the output of the following program?
1: public class FeedingSchedule {
2: public static void main(String[] args) {
3: int x = 5, j = 0;
4: OUTER: for(int i=0; i<3; )
5: INNER: do {
6: i++; x++;
7: if(x > 10) break INNER;
8: x += 4;
9: j++;
10: } while(j <= 2);
11: System.out.println(x);
12: } } - What is the result of the following program?
1: public class Egret {
2: private String color;
3: public Egret() {
4: this(“white”);
5: }
6: public Egret(String color) {
7: color = color;
8: }
9: public static void main(String[] args) {
10: Egret e = new Egret();
11: System.out.println(“Color:” + e.color);
12: }
13: } - What is the output of the following program?
1: public class BearOrShark {
2: public static void main(String[] args) {
3: int luck = 10;
4: if((luck>10 ? luck++: –luck)<10) {
5: System.out.print(“Bear”);
6: } if(luck<10) System.out.print(“Shark”);
7: } } - Assuming we have a valid, non-null HenHouse object whose value is initialized by the
blank line shown here, which of the following are possible outputs of this application?
1: class Chicken {}
2: interface HenHouse { public java.util.List<Chicken> getChickens(); }
3: public class ChickenSong {
4: public static void main(String[] args) {
5: HenHouse house = ______________
6: Chicken chicken = house.getChickens().get(0);
7: for(int i=0; i<house.getChickens().size();
8: chicken = house.getChickens().get(i++)) {
9: System.out.println(“Cluck”);
10: } } } - Which of the following statements can be inserted in the blank line so that the code will
compile successfully?
public interface CanSwim {}
public class Amphibian implements CanSwim {}
class Tadpole extends Amphibian {}
public class FindAllTadPole {
public static void main(String[] args) {
List<Tadpole> tadpoles = new ArrayList<Tadpole>();
for(Amphibian amphibian : tadpoles) {
___________ tadpole = amphibian;
} } } - What individual changes, if any, would allow the following code to compile?
1: public interface Animal { public default String getName() { return null; } }
2: interface Mammal { public default String getName() { return null; } }
3: abstract class Otter implements Mammal, Animal {}- Which of the following lines can be inserted at line 11 to print true?
1: public static void main(String[] args) {
2: // INSERT CODE HERE
3: }
4: private static boolean test(Predicate<Integer> p) {
5: return p.test(5);
6: }- What is the print out a date representing April 1, 2015?
- Bytecode is in a file with which extension?
- Name any two checked exceptions?
- What are the OOP features?
- What is Abstraction?
- What is Encapsulation?
- What is Inheritance?
- What is Polymorphism?
- What is Object?
- What is a Constructor?
- What is meant by Local variable and Instance variable?
- What is meant by Method Overriding?
- What is meant by Overloading?
- What is meant by Interface?
- Difference between Abstract class and Interface.
- What is meant by Exception?
- What are the types of Exceptions?
- Explain about Exception Propagation.
- What is the final keyword in Java?
- What is a Thread?
- How do you make a thread in Java?
- How are Java objects stored in memory?
- What is static variable in Java?
- What is object cloning?
- Why is String Immutable in Java?
- What is the size of int in 64-bit JVM?
- What is the difference between JRE, JDK, JVM and JIT?
- What’s the difference between “a == b” and “a.equals(b)”?
- What is a hashCode() used for? How is it related to a.equals(b)?
- Difference between final, finalize and finally?
ANSWERS @https://ipwithease.com/shop/
Check our new website https://networkinterview.com For Free Video Courses, Technopedia, Mindmaps, Cheatsheets and much more.
ABOUT THE AUTHOR
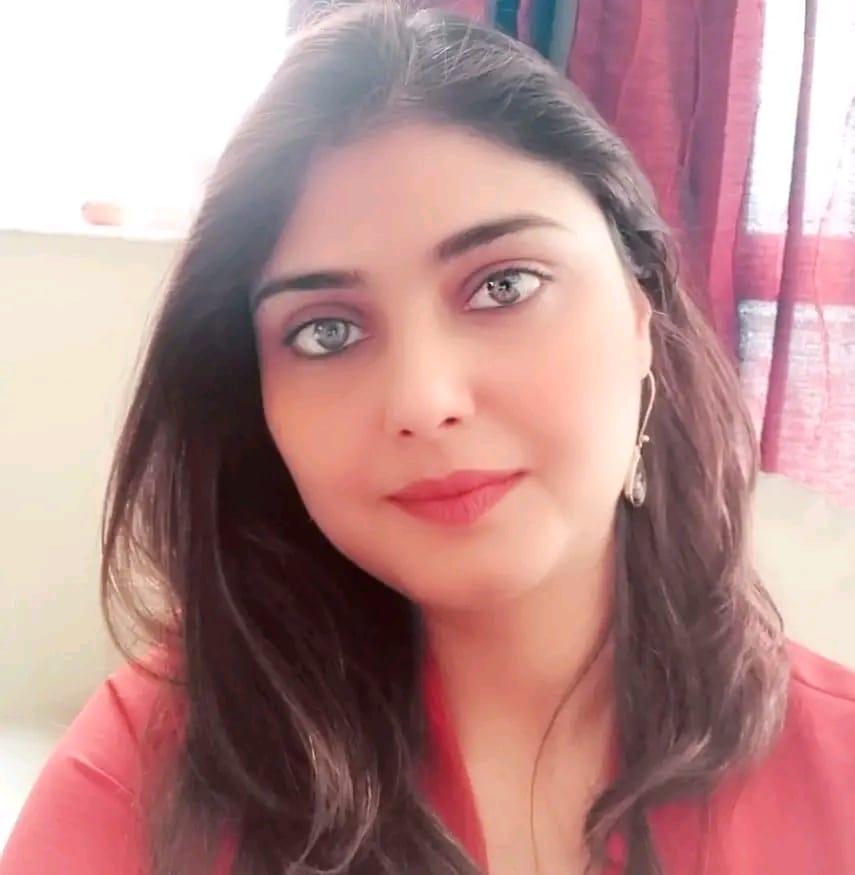
Founder of AAR TECHNOSOLUTIONS, Rashmi is an evangelist for IT and technology. With more than 12 years in the IT ecosystem, she has been supporting multi domain functions across IT & consultancy services, in addition to Technical content making.
You can learn more about her on her linkedin profile – Rashmi Bhardwaj