Table of Contents
To push and remove configurations on network devices using pyATS (Python Automation Test System), you’ll typically leverage pyATS’s Unicon library to interact with the device’s CLI.
In this blog, we will learn to how to use pyATS for pushing and removing configurations.
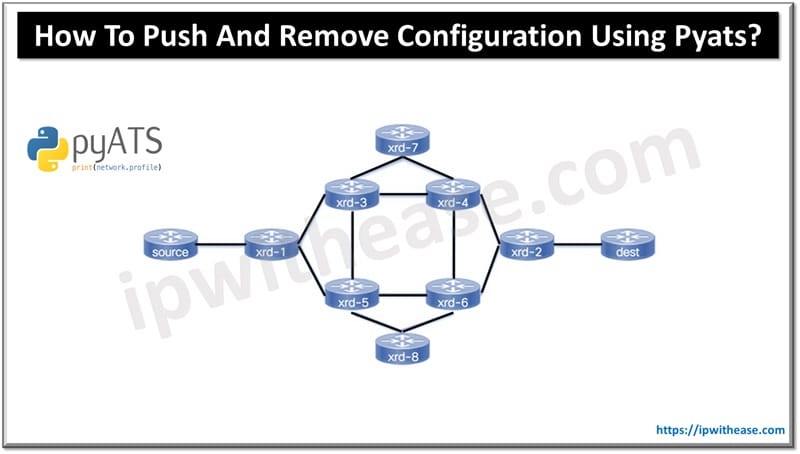
Push and Remove Configuration using pyATS
Prerequisites
1.pyATS and Unicon installed. (Steps to Install pyATS )
pip install pyats[full] unicon
2.Device connection credentials available in a testbed.yaml file or directly in the code.
Step 1: Create the Testbed
Set up a testbed file in YAML format, which defines your devices and access credentials:
testbed:
name: 'sample_testbed'
devices:
router1:
os: 'iosxe'
type: 'router'
connections:
cli:
protocol: 'ssh'
ip: '192.168.1.1'
credentials:
default:
username: 'your_username'
password: 'your_password'
Step 2: Establish Connection with the Device
Use pyATS to connect to the device and push configurations. Below is an example to add and remove configuration using Unicon:
Code to Push and Remove Configurations
from genie.testbed import load
from unicon.core.errors import SubCommandFailure
# Load the testbed
testbed = load('testbed.yaml')
# Select the device
device = testbed.devices['router1']
try:
# Connect to the device
device.connect()
# Define configuration commands to add
config_commands = [
'interface GigabitEthernet1',
'description Connected to LAN',
'ip address 192.168.10.1 255.255.255.0',
'no shutdown'
]
# Push configuration
device.configure(config_commands)
print("Configuration applied successfully.")
# Removing configuration (e.g., remove IP address and description)
remove_commands = [
'interface GigabitEthernet1',
'no description',
'no ip address'
]
# Remove configuration
device.configure(remove_commands)
print("Configuration removed successfully.")
except SubCommandFailure as e:
print(f"Configuration failed: {e}")
finally:
# Disconnect from the device
device.disconnect()
Explanation of the Code
- Connect to the Device: The device.connect() function establishes a connection using the details in your testbed.yaml.
- Push Configuration: The device.configure(config_commands) method applies the list of configuration commands to the device.
- Remove Configuration: Using device.configure(remove_commands), you send the commands to remove configurations (such as removing an IP address or description).
- Error Handling: If a command fails, SubCommandFailure will catch the error.
- Disconnect: The device.disconnect() closes the connection.
Additional Tips
- Ensure the testbed.yaml is correctly defined for other devices if needed.
- For more complex configurations or templated setups, consider using Jinja templates to streamline the configurations you apply.
This method allows for quick, scriptable configuration management across network devices using pyATS.
Related FAQs
Q.1 What should I do if pyATS fails to connect to a device?
- Ensure the IP address and protocol in the
testbed
file are correct. - Verify the device credentials.
- Confirm that the device allows the specified connection protocol (e.g., SSH).
Q.2 How do I troubleshoot ConnectionRefusedError in pyATS?
Verify the following:
- The device is reachable (ping it from your host).
- Correct credentials and protocol in the testbed.
- Appropriate SSH/Telnet settings are enabled on the device.
Q.3 How can I handle timeouts during pyATS connections?
Increase the timeout in the testbed
file under the connection
parameters:
connections:
cli:
protocol: ssh
ip: 192.168.1.1
timeout: 60 # Increase timeout
Q.4 Can pyATS work with non-Cisco devices?
Yes, pyATS supports multiple vendors by using generic APIs or developing custom parsers for specific devices.
Q.5 How do I add new libraries or dependencies to my pyATS environment?
Use pip to install any additional packages:
pip install <package-name>
Q.6 How do I verify if pyATS is installed correctly?
Run the following command:
pyats version
Q.7 What is the role of a testbed file in pyATS?
The testbed file is a YAML or JSON file that defines the topology and credentials of network devices. It acts as the primary configuration file for pyATS tests.
Q.8 What does DeviceNotFoundError mean?
This error occurs when the specified device in the script is not found in the testbed
file. Ensure the device name matches exactly.
Q.9 How do I resolve ModuleNotFoundError in pyATS?
Install the missing module using pip:
pip install <module-name>
Q.10 How can I debug failed test cases?
Enable debug mode by adding –debug to your command:
pyats run job sample_job.py --debug
ABOUT THE AUTHOR
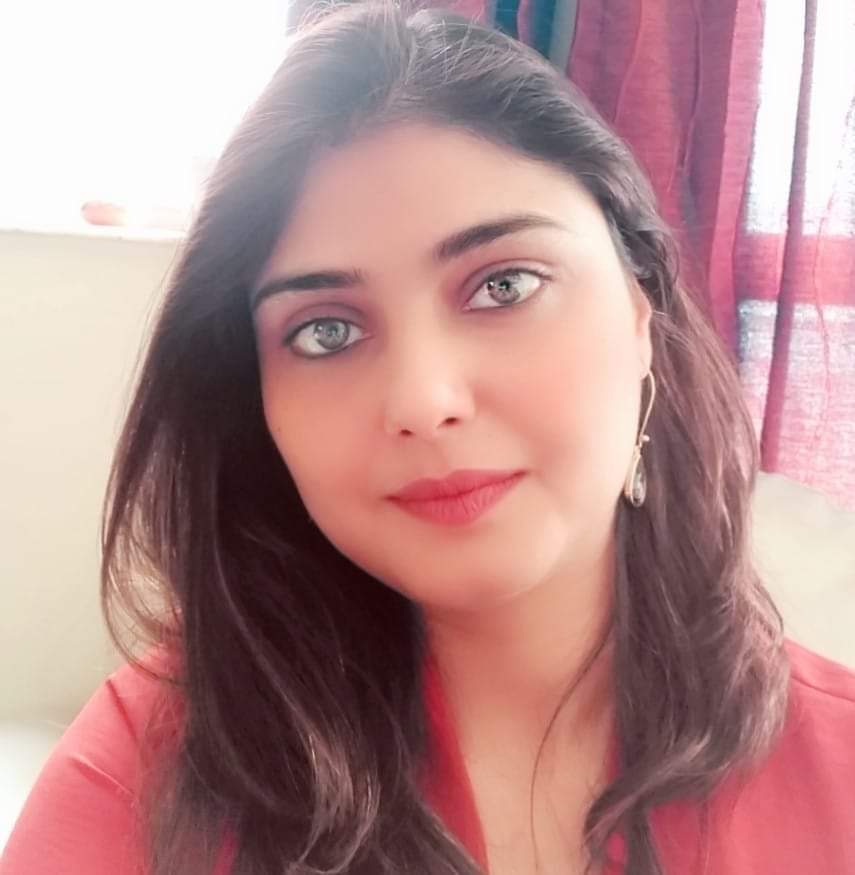
I am here to share my knowledge and experience in the field of networking with the goal being – “The more you share, the more you learn.”
I am a biotechnologist by qualification and a Network Enthusiast by interest. I developed interest in networking being in the company of a passionate Network Professional, my husband.
I am a strong believer of the fact that “learning is a constant process of discovering yourself.”
– Rashmi Bhardwaj (Author/Editor)